BOSCH BMP180 Atmospheric Temperature / Pressure Sensor Module
This board is 5V compliant - a 3.3V regulator and a i2c level shifter circuit is included so you can use this sensor safely with 5V logic and power.
Using the sensor is easy. For example, if you're using an Arduino, simply connect the VIN pin to the 5V voltage pin, GND to ground, SCL to I2C Clock (Analog 5) and SDA to I2C Data (Analog 4). Then download our BMP085/BMP180 Arduino library and example code for temperature, pressure and altitude calculation. Install the library, and load the example sketch. Immediately you'll have precision temperature, pressure and altitude data. Our detailed tutorial has all the info you need including links to software and installation instructions. It includes more information about the BMP180 so you can understand the sensor in depth including how to properly calculate altitude based on sea-level barometric pressure.
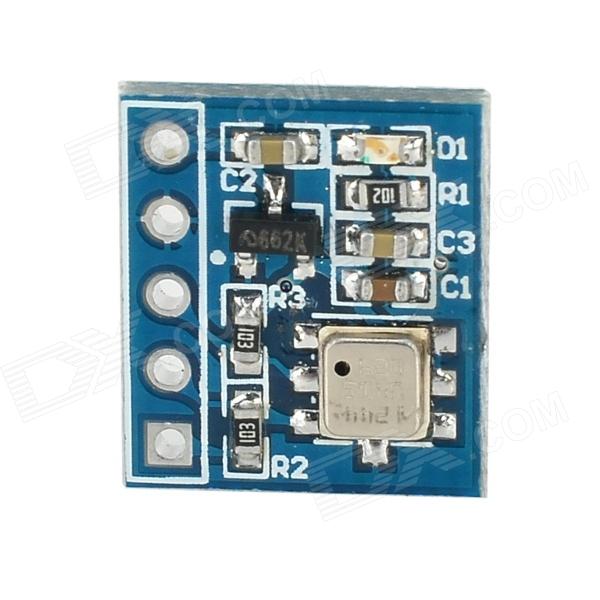 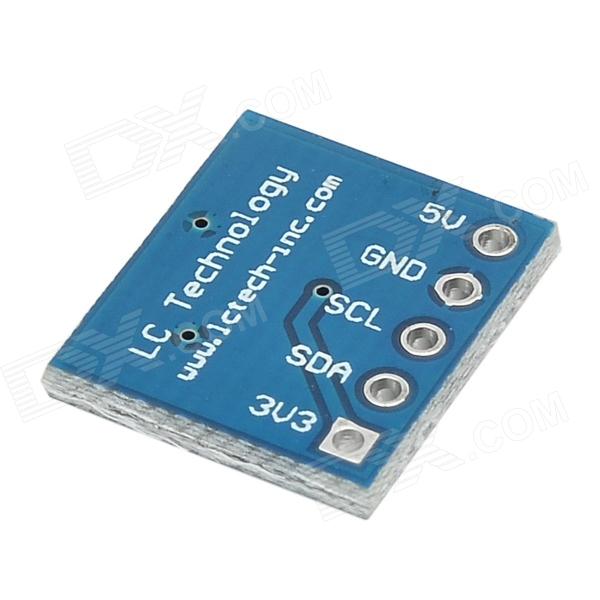
The BMP085 is a basic sensor that is designed specifically for measuring barometric pressure (it also does temperature measurement on the side to help). It's one of the few sensors that does this measurement, and its fairly low cost so you'll see it used a lot. You may be wondering why someone would want to measure atmospheric pressure, but its actually really useful for two things. One is to measure altitude. As we travel from below sea level to a high mountain, the air pressure decreases. That means that if we measure the pressure we can determine our altitude - handy when we don't want the expense or size of a GPS unit. Secondly, atmospheric pressure can be used as a predictor of weather which is why weather-casters often talk about "pressure systems"
Specifications
-
Pressure sensing range: 300-1100 hPa (9000m to -500m above sea level)
-
Up to 0.03hPa / 0.25m resolution
-
-40 to +85°C operational range, +-2°C temperature accuracy
-
2-pin i2c interface on chip
-
V1 of the breakout uses 3.3V power and logic level only
-
V2 of the breakout uses 3.3-5V power and logic level for more flexible usage
Wiring the BMP085
Since the BMP085 is a i2c sensor, its very easy to wire up. We'll be using an Arduino as an example but any microcontroller with i2c can be used.
Connect the VCC pin to a 3.3V power source. The V1 of the sensor breakout cannot be used with anything higher than 3.3V so don't use a 5V supply! V2 of the sensor board has a 3.3V regulator so you can connect it to either 3.3V or 5V if you do not have 3V available.
Connect GND to the ground pin.
Connect the i2c SCL clock pin to your i2c clock pin. On the classic Arduino Uno/Duemilanove/Diecimila/etc this is Analog pin #5
Connect the i2c SDA data pin to your i2c data pin. On the classic Arduino Uno/Duemilanove/Diecimila/etc this is Analog pin #4
Unfortunately, the i2c lines on most microcontrollers are fixed so you're going to have to stick with those pins.
You don't need to connect the XCLR (reset) or EOC (end-of-conversion) pins. If you need to speed up your conversion time, you can use the EOC as a indicator - in our code we just hang out and wait the maximum time possible.
Using the BMP085 (API v2)
This page is based on the new v2 of the BMP085 driver, which uses Adafruit's new Unified Sensor Driver. The driver provides better support for altitude calculations, and makes it easy to switch between the BMP085 and any other supported pressure sensor in your projects.
If you haven't already done so, you'll need to install the Adafrut_Sensor library on your system as well, since Adafruit_BMP085 relies on this library to generate the sensor data in a universal manner.
To use this sensor and calculate the altitude and barometric pressure, there's a lot of very hairy and unpleasant math. You can check out the math in the datasheet but really, its not intuitive or educational - its just how the sensor works. So we took care of all the icky math and wrapped it up into a nice Arduino library.
Install the library Adafruit_Sensor-master, Adafruit_BMP085_Unified-master.
Now you can run this first example sketch
#include
#include
#include
Adafruit_BMP085_Unified bmp = Adafruit_BMP085_Unified(10085);
void setup(void)
{
Serial.begin(9600);
Serial.println("Pressure Sensor Test"); Serial.println("");
/* Initialise the sensor */
if(!bmp.begin())
{
/* There was a problem detecting the BMP085 ... check your connections */
Serial.print("Ooops, no BMP085 detected ... Check your wiring or I2C ADDR!");
while(1);
}
}
void loop(void)
{
/* Get a new sensor event */
sensors_event_t event;
bmp.getEvent(&event);
/* Display the results (barometric pressure is measure in hPa) */
if (event.pressure)
{
/* Display atmospheric pressure in hPa */
Serial.print("Pressure: "); Serial.print(event.pressure); Serial.println(" hPa");
}
else
{
Serial.println("Sensor error");
}
delay(250);
}
Then open up the serial monitor at 9600 baud. The sketch will continuously print out the pressure in hPa (hectoPascals). You can test that the sensor is measuring variations in pressure by placing your fingertip over the open port hole in the top of the sensor. The pressure will increase as you can see here:
Altitude Measurements
update the 'void loop()' function above with the code below to get the altitude based on the pressure and temperature:
void loop(void)
{
/* Get a new sensor event */
sensors_event_t event;
bmp.getEvent(&event);
/* Display the results (barometric pressure is measure in hPa) */
if (event.pressure)
{
/* Display atmospheric pressue in hPa */
Serial.print("Pressure: ");
Serial.print(event.pressure);
Serial.println(" hPa");
/* Calculating altitude with reasonable accuracy requires pressure *
* sea level pressure for your position at the moment the data is *
* converted, as well as the ambient temperature in degress *
* celcius. If you don't have these values, a 'generic' value of *
* 1013.25 hPa can be used (defined as SENSORS_PRESSURE_SEALEVELHPA *
* in sensors.h), but this isn't ideal and will give variable *
* results from one day to the next. *
* *
* You can usually find the current SLP value by looking at weather *
* websites or from environmental information centers near any major *
* airport. *
* *
* For example, for Paris, France you can check the current mean *
* pressure and sea level at: http://bit.ly/16Au8ol */
/* First we get the current temperature from the BMP085 */
float temperature;
bmp.getTemperature(&temperature);
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(" C");
/* Then convert the atmospheric pressure, SLP and temp to altitude */
/* Update this next line with the current SLP for better results */
float seaLevelPressure = SENSORS_PRESSURE_SEALEVELHPA;
Serial.print("Altitude: ");
Serial.print(bmp.pressureToAltitude(seaLevelPressure,
event.pressure,
temperature));
Serial.println(" m");
Serial.println("");
}
else
{
Serial.println("Sensor error");
}
delay(1000);
}
Run the sketch to see the calculated altitude.
The data above is reasonably close to what I'd expect at my location, but we can improve the accuracy by changing the reference sea level pressure, which will change depending on the weather conditions. Every 1 hPa that we are off on the sea level pressure equals about 8.5 m of error in the altitude calculations!
Url:
BMP085_DataSheet_Rev.1.0_01July2008
Adafruit_Sensor-master
Adafruit_BMP085_Unified-master Library v2
Adafruit-BMP085-Library-master Library v1
ref:https://www.adafruit.com/
http://www.dx.com
https://github.com/adafruit/adafruit-bmp085-library
|